VMscheduling is a critical component in cloud computing simulations, determining how computational resources like CPU, memory, and bandwidth are allocated to VMs running on physical hosts.
In CloudSim, a powerful simulation toolkit for cloud computing, VMscheduling plays a central role in managing resource allocation and optimizing performance.
This blog post introduces the class hierarchy of the VmScheduler class in CloudSim and provides a step-by-step guide to implementing your own custom scheduling policy.
If you’re a beginner or intermediate user of CloudSim, this guide will help you deepen your understanding and take your simulations to the next level.
What is VMScheduling?
In the context of CloudSim, VMscheduling is the process of deciding how resources on a host are distributed among multiple VMs.
Different scheduling policies can be applied based on the workload, objectives (e.g., fairness, performance, or energy efficiency), and resource constraints.
Key Components of VMScheduling in CloudSim
CloudSim provides a base class called VmScheduler that defines the general structure and behaviour for VMscheduling.
From this base class, several predefined scheduling policies are implemented, each tailored to specific scenarios.
Class Hierarchy of VmScheduler
Here’s a visual representation of the VmScheduler class hierarchy:
- VmScheduler: The abstract base class for VMscheduling.
- VmSchedulerTimeShared: Allocates CPU in time slices across multiple VMs.
- VmSchedulerSpaceShared: Allocates all resources to one VM at a time.
- VmSchedulerTimeSharedOverSubscription: Simulates resource over-subscription, where VMs are allocated more resources than the host physically provides.
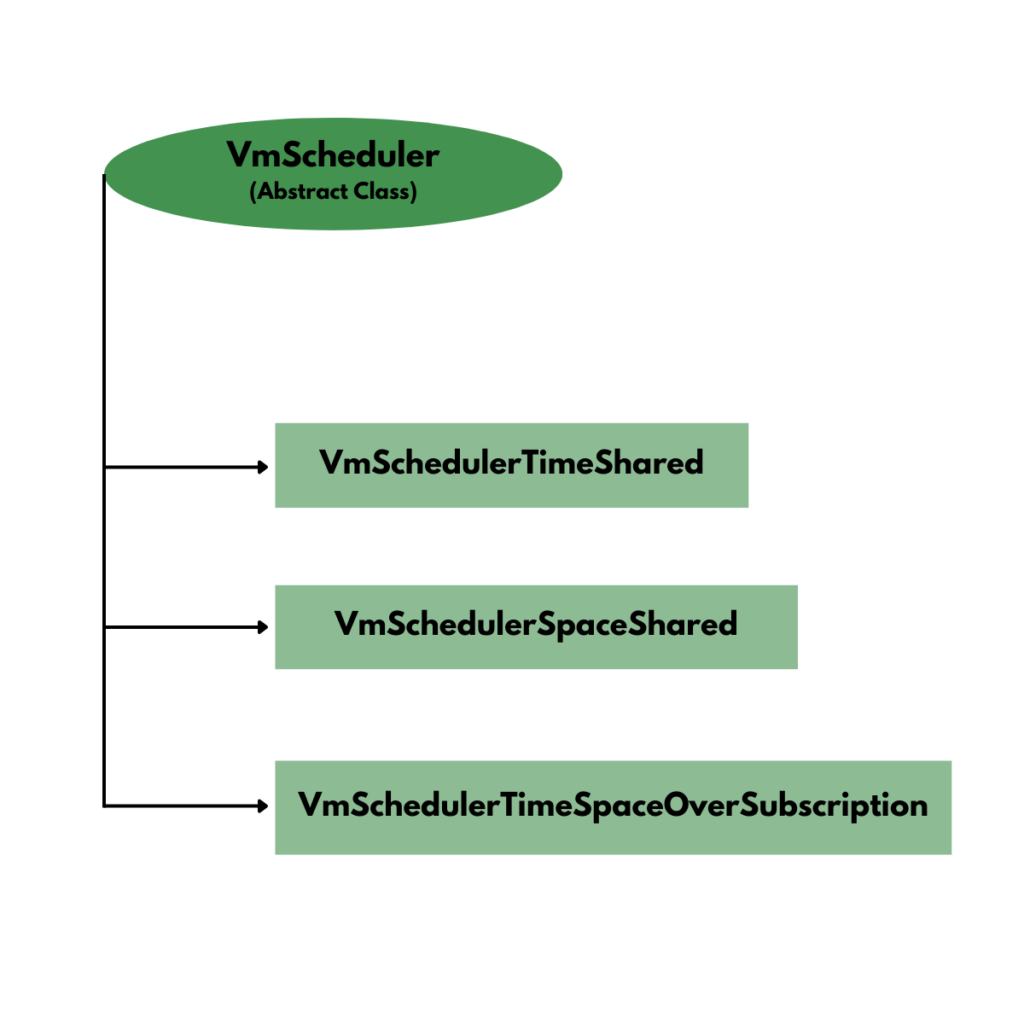
Each of these policies has unique characteristics, making them suitable for different simulation needs.
How Does VMScheduling Work?
The VmScheduler class manages:
- Allocation of Processing Elements (PEs): CPU resources are divided into smaller units called PEs.
- Deallocation of Resources: Frees resources when a VM is no longer active.
- Resource Management: Ensures fair and efficient distribution of resources among VMs.
Key methods in the VmScheduler class include:
- allocatePesForVm(Vm vm, List mipsShare): Allocates resources to a VM.
- deallocatePesForVm(Vm vm): Frees resources allocated to a VM.
- getAvailableMips(): Returns the remaining MIPS (Million Instructions Per Second) available on the host.
Predefined Scheduling Policies in CloudSim
- VmSchedulerTimeShared:
- Resources are shared among all VMs in time slices.
- Ideal for scenarios requiring fairness.
- Example: A VM uses CPU resources for a limited time before passing control to another VM.
- VmSchedulerSpaceShared:
- Allocates all resources of a host to one VM at a time.
- Suitable for dedicated environments.
- Example: One VM runs to completion before another VM is allocated resources.
- VmSchedulerTimeSharedOverSubscription:
- Allows over-provisioning of resources to simulate contention.
- Useful for understanding how systems behave under resource constraints.
Creating Your Own VMScheduling Policy
You can extend the VmScheduler class to create a custom scheduling policy. Here’s how:
1. Extend the VmScheduler Class:
public class CustomVmScheduler extends VmScheduler {
public CustomVmScheduler(List<? extends Pe> pelist) {
super(pelist);
}
@Override
public boolean allocatePesForVm(Vm vm, List<Double> mipsShare) {
// Custom logic for resource allocation
return super.allocatePesForVm(vm, mipsShare);
}
@Override
public void deallocatePesForVm(Vm vm) {
// Custom logic for resource deallocation
super.deallocatePesForVm(vm);
}
}
2. Define Scheduling Logic:
Implement your custom logic in the allocatePesForVm method. For example:
- A priority-based scheduler can allocate more resources to higher-priority VMs.
- A weighted scheduler can allocate resources based on predefined weights.
3. Integrate Your Scheduler:
Assign your custom scheduler to a host in your simulation:
Host host = new Host(
id,
new RamProvisionerSimple(ram),
new BwProvisionerSimple(bw),
storage,
peList,
new CustomVmScheduler(peList)
);
4. Run Simulations:
Use your custom scheduler in a simulation to observe its impact on resource allocation and performance.
Practical Example: Priority-Based Scheduler
Here’s an example of a custom scheduler that prioritizes VMs:
public class PriorityVmScheduler extends VmScheduler {
private Map<Vm, Integer> vmPriorityMap;
public PriorityVmScheduler(List<? extends Pe> pelist) {
super(pelist);
vmPriorityMap = new HashMap<>();
}
public void setVmPriority(Vm vm, int priority) {
vmPriorityMap.put(vm, priority);
}
@Override
public boolean allocatePesForVm(Vm vm, List<Double> mipsShare) {
int priority = vmPriorityMap.getOrDefault(vm, 0);
if (priority > 5) {
return super.allocatePesForVm(vm, mipsShare.stream().map(mips -> mips * 1.5).toList());
}
return super.allocatePesForVm(vm, mipsShare);
}
}
This scheduler allocates more resources to high-priority VMs by adjusting the MIPS share.
Conclusion
Understanding VMscheduling in CloudSim is crucial for effectively simulating cloud environments.
By exploring the predefined scheduling policies and creating your own, you can gain deeper insights into resource allocation and optimization.
This knowledge is invaluable for researchers and developers aiming to solve real-world problems in cloud computing.
Ready to take your CloudSim skills to the next level?
Start experimenting with custom policies and share your results in the comments below.
Interested in learning more?
Join my course community at SuperWitsAcademy.online for in-depth tutorials and resources on CloudSim and cloud computing research.
Happy simulating!